Docker. Reminder
Nowadays it's fashionable to put everything in containers. It's like virtual machines but not really. Legends circulate in the forest that if you put all applications in containers, security, universal happiness, and even communism will arrive. So I tried to wrap various things in Docker. And to not forget, I'm writing this reminder.
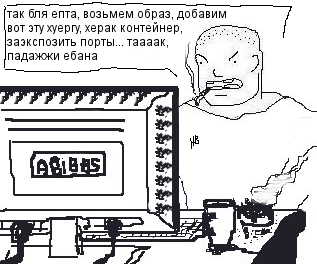
Installing Docker
In your favorite distribution "install docker". For my favorite Fedora:
sudo dnf install docker
Start the daemon, and enable autostart:
sudo systemctl start docker
sudo systemctl enable docker
Check that everything works
sudo docker run hello-world
Various letters should appear. If they appear, ok, if not, decide for yourself here's the manual
Now, to avoid pestering sudo unnecessarily, let's add ourselves to the group.
sudo groupadd docker
sudo usermod -aG docker $USER
Log out, log in, you can also restart the daemon? Check.
docker run hello-world
Didn't work? Do it like a noob, reboot the machine!
Images
In Docker there are several simple entities: image, container, volume, network. The rest isn't important yet.
Image, it's an image in which some Linux is "baked" and on top of it everything that you or someone else stuffed in. Like an ISO.
- View a list of all images currently on your machine:
docker images
- Download some image from space:
docker pull <image name>
- You can also download a specific version:
docker pull <image name>:<version, also known as tag>
> - Delete an image:
docker rmi <image name>
Containers
Containers are such very isolated things that are obtained when an image is "unfolded into memory and launched". Imagine a virtual machine image from which you can create a thousand running instances.
- Running containers
docker ps
- All containers
docker ps -a
- Create and run a container
docker run --name=<container name> <image name>
- Start an already created container
docker start <container name>
- Stop a container
docker stop <container name>
- Delete a container
docker rm <container name>
There are also a couple of useful keys for launch:
--detach, -d, --interactive, -i, --tty, -t and --publish, -p
The --name parameter can be omitted, Docker will assign some funny name like thirsty_bison. The name can be used later as the hostname within the network.
Networks
All containers live in isolated networks. This is not a virtual machine on a bridge!
- List of networks
docker network ls
- Create a network
docker network create <network name>
- Delete a network
docker network rm <network name>
- Specify a network for a container at launch
docker run --network=<network name> <image name>
Networks are very convenient to use so that containers can somehow communicate with each other. For example, if you have a reverse proxy and 2 WordPress sites. Add them all to one network. Then, you can use the container name as the hostname, for example in the reverse proxy config. In old manuals, the --link key was used for this, now it's outdated and not fashionable at all.
Volume or "Volume?"
Containers are practically immutable entities, almost read-only. And if you stop the container, the info is gone. And if you need to save something, it's convenient to use volumes. This is a thing to mount something from the host's file system, where Docker is running, to the container's file system. For example, create and run an nginx container with a config that lies somewhere on the host:
docker run -v /somepath/nginx.conf:/etc/nginx/nginx.conf -p 80:80 nginx
There's a moment with SELinux. If the container can't start, and you specified the paths correctly, try using the :z suffix, this will set the SELinux labels for this path as needed:
docker run -v /somepath/nginx.conf:/etc/nginx/nginx.conf:z -p 80:80 nginx
- View a list of all volumes
docker volume ls
- Create a volume
docker volume create <volume name>
- Create and run a container, automatically creating and mounting a volume
docker run -v <volume name>:/var/www <image name>
Volumes for the host, practically folders, usually lie here /var/lib/docker/volumes
Logs
Often it happens that a container starts and immediately stops because of an error. You can view the logs with:
docker logs <container name>
Creating your own images
And finally, you can create your own images. For this, create some folder, and in it a file Dockerfile. There are several directives.
FROM - from which image we'll start "layering" our own.
RUN - run a command, for example yum update
COPY <what> <where> - copy a file. The first parameter is the path to the file relative to the current folder on the host. The second, the path inside the image. It's important not to mess up.
I, for example, inattentively when creating a new image wrote:
COPY nginx.conf /etc/nginx/nginx.conf;
And for a long time couldn't understand why in the running container there was a default /etc/nginx/nginx.conf, and not the one that should have been copied. Because the semicolon here is fucking unnecessary and Docker did everything correctly, copied my nginx.conf to /etc/nginx/nginx.conf; and nginx was reading /etc/nginx/nginx.conf Like that.
And finally, there's the inspect command, very convenient to see how a container, network, or volume is configured.
docker inspect <container name>
docker network inspect <network name>
docker volume inspect <volume name>
If you want to understand deeper, go read the tutorial